In this article, I will demonstrate about Angular JS CRUD operations example with Web API. Web API will be used as a service to get the data from database and share the data to angular js app. For this demonstration, I have used Code First Approach.
In this example, I will show you how to make routing in AngularJS and perform CRUD [Create, Read, Update and Delete] Operations. All the operations will be perform with different template page [html page].
Create Asp.Net Application
To create new application, open Visual Studio 2015 and Select File | New and then Project. It will open a New Project window, choose Web tab inside Visual C# and then choose Asp.Net Web Application. You can choose your project location and click to OK.
From the New Asp.Net Project window, just choose Web API project and click to OK.
Create Model and Context Classes
Following is the employee model, where all the properties have defined for employee. I have used Table attribute for table name.
using System;
using System.ComponentModel.DataAnnotations;
using System.ComponentModel.DataAnnotations.Schema;
namespace CRUDwithAngularJSAndWebAPI.Models
{
[Table("Employees")]
public class EmployeeModel
{
[Key]
public int EmployeeID { get; set; }
[Required(ErrorMessage ="First Name Required")]
[StringLength(maximumLength:20, MinimumLength =3, ErrorMessage ="Name should be between 3 to 20 characters")]
public string FirstName { get; set; }
public string LastName { get; set; }
public string Address { get; set; }
public float Salary { get; set; }
public DateTime DOB { get; set; }
}
}
Following is the Database access context class where I have defined the database connection.
using System.Data.Entity;
namespace CRUDwithAngularJSAndWebAPI.Models
{
public class DataAccessContext : DbContext
{
public DataAccessContext() : base("testconnection")
{
}
public DbSet<EmployeeModel> Employees { get; set; }
}
}
Create API Controller
As we are using Web API to access data from database. It will required to create controller to access the data. To create new API controller. Right click on Controller folder choose Add and then select Controller. You need to provide the controller name as "EmployeeController".
Make the code changes in EmployeeController as following.
Here I have made the code for Create, Read, Update and Delete. Don't forget to assign the request type with action method.
using CRUDwithAngularJSAndWebAPI.Models;
using CRUDwithAngularJSAndWebAPI.ViewModel;
using System;
using System.Collections.Generic;
using System.Globalization;
using System.Linq;
using System.Net;
using System.Net.Http;
using System.Web.Http;
namespace CRUDwithAngularJSAndWebAPI.Controllers
{
public class EmployeeController : ApiController
{
DataAccessContext context = new DataAccessContext();
//Get All Employees
[HttpGet]
public IEnumerable<EmployeeViewModel> GetAllEmployee()
{
var data = context.Employees.ToList().OrderBy(x=>x.FirstName);
var result = data.Select(x => new EmployeeViewModel()
{
EmployeeID = x.EmployeeID,
FirstName = x.FirstName,
LastName = x.LastName,
Address = x.Address,
Salary = x.Salary,
DOB = x.DOB
});
return result.ToList();
}
//Get the single employee data
[HttpGet]
public EmployeeViewModel GetEmployee(int Id)
{
var data = context.Employees.Where(x => x.EmployeeID == Id).FirstOrDefault();
if (data != null)
{
EmployeeViewModel employee = new EmployeeViewModel();
employee.EmployeeID = data.EmployeeID;
employee.FirstName = data.FirstName;
employee.LastName = data.LastName;
employee.Address = data.Address;
employee.Salary = data.Salary;
employee.DOB = data.DOB;
return employee;
}
else
{
throw new HttpResponseException(Request.CreateResponse(HttpStatusCode.NotFound));
}
}
//Add new employee
[HttpPost]
public HttpResponseMessage AddEmployee(EmployeeViewModel model)
{
try
{
if (ModelState.IsValid)
{
EmployeeModel emp = new EmployeeModel();
emp.FirstName = model.FirstName;
emp.LastName = model.LastName;
emp.Address = model.Address;
emp.Salary = model.Salary;
emp.DOB = Convert.ToDateTime(model.DOB.ToString("yyyy-MM-dd HH:mm:ss.fff"));
context.Employees.Add(emp);
var result = context.SaveChanges();
if (result > 0)
{
return Request.CreateResponse(HttpStatusCode.Created, "Submitted Successfully");
}
else
{
return Request.CreateErrorResponse(HttpStatusCode.BadRequest, "Something wrong !");
}
}
else
{
return Request.CreateErrorResponse(HttpStatusCode.BadRequest, "Something wrong !");
}
}
catch (Exception ex)
{
return Request.CreateErrorResponse(HttpStatusCode.BadRequest, "Something wrong !", ex);
}
}
//Update the employee
[HttpPut]
public HttpResponseMessage UpdateEmployee(EmployeeViewModel model)
{
try
{
if (ModelState.IsValid)
{
EmployeeModel emp = new EmployeeModel();
emp.EmployeeID = model.EmployeeID;
emp.FirstName = model.FirstName;
emp.LastName = model.LastName;
emp.Address = model.Address;
emp.Salary = model.Salary;
emp.DOB = Convert.ToDateTime(model.DOB.ToString("yyyy-MM-dd HH:mm:ss.fff"));
context.Entry(emp).State = System.Data.Entity.EntityState.Modified;
var result = context.SaveChanges();
if (result > 0)
{
return Request.CreateResponse(HttpStatusCode.OK, "Updated Successfully");
}
else
{
return Request.CreateErrorResponse(HttpStatusCode.NotFound, "Something wrong !");
}
}
else
{
return Request.CreateErrorResponse(HttpStatusCode.BadRequest, "Something wrong !");
}
}
catch (Exception ex)
{
return Request.CreateErrorResponse(HttpStatusCode.BadRequest, "Something wrong !", ex);
}
}
//Delete the employee
[HttpDelete]
public HttpResponseMessage DeleteEmployee(int Id)
{
EmployeeModel emp = new EmployeeModel();
emp = context.Employees.Find(Id);
if (emp != null)
{
context.Employees.Remove(emp);
context.SaveChanges();
return Request.CreateResponse(HttpStatusCode.OK, emp);
}
else
{
return Request.CreateErrorResponse(HttpStatusCode.NotFound, "Something wrong !");
}
}
}
}
As you can see wtih above code I have used a ViewModel, It is becasue in future if you want to extend the functionality with relational data, there will not be long change. View Model is used because of merging the multiple tables data.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace CRUDwithAngularJSAndWebAPI.ViewModel
{
public class EmployeeViewModel
{
public int EmployeeID { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public string Address { get; set; }
public float Salary { get; set; }
public DateTime DOB { get; set; }
//Following are the extend properties if you want
public int DepartmentID { get; set; }
public string DepartmentName { get; set; }
}
}
Create Connection String
Create connection string insdie the web.config and call it with database context class.Please make sure change the user name and password when running the application on your system.
<connectionStrings>
<add name="testconnection" connectionString="Data Source=my-computer; database = DemoTest; user id = mukesh; password =adminadmin;" providerName="System.Data.SqlClient" />
</connectionStrings>
Create Angular JS App
Now it is time to create angular js [Single Page Application] in shore SPA. Here we will be used API service to perform CRUD operations using angular js. Create a folder named with "App" and inside this folder create two JS files like "app.js" and "EmployeeController.js".
You need to create Views folder inside the App folder and create 4 html template files for CRUD operations as following image.
App.Js
As we know, an Angular JS application run inside the ng-app directive. So, first step to create routing for angular js app.
var app = angular.module('myApp', ['ngRoute']);
app.config(['$locationProvider', '$routeProvider', function ($locationProvider, $routeProvider) {
$routeProvider.when('/EmployeeList', { //Routing for show list of employee
templateUrl: '/App/Views/EmployeeList.html',
controller: 'EmployeeController'
}).when('/AddEmployee', { //Routing for add employee
templateUrl: '/App/Views/AddEmployee.html',
controller: 'EmployeeController'
})
.when('/EditEmployee/:empId', { //Routing for geting single employee details
templateUrl: '/App/Views/EditEmployee.html',
controller: 'EmployeeController'
})
.when('/DeleteEmployee/:empId', { //Routing for delete employee
templateUrl: '/App/Views/DeleteEmployee.html',
controller: 'EmployeeController'
})
.otherwise({ //Default Routing
controller: 'EmployeeController'
})
}]);
EmployeeController.js
Now to perform CRUD operations on client side, we need to add EmployeeController.js file which will hanlde all the CRUD opertions with http services.
app.controller("EmployeeController", ['$scope', '$http', '$location', '$routeParams', function ($scope, $http, $location, $routeParams) {
$scope.ListOfEmployee;
$scope.Status;
$scope.Close = function () {
$location.path('/EmployeeList');
}
//Get all employee and bind with html table
$http.get("api/employee/GetAllEmployee").success(function (data) {
$scope.ListOfEmployee = data;
})
.error(function (data) {
$scope.Status = "data not found";
});
//Add new employee
$scope.Add = function () {
var employeeData = {
FirstName: $scope.FirstName,
LastName: $scope.LastName,
Address: $scope.Address,
Salary: $scope.Salary,
DOB: $scope.DOB,
// DepartmentID: $scope.DepartmentID
};
debugger;
$http.post("api/employee/AddEmployee", employeeData).success(function (data) {
$location.path('/EmployeeList');
}).error(function (data) {
console.log(data);
$scope.error = "Something wrong when adding new employee " + data.ExceptionMessage;
});
}
//Fill the employee records for update
if ($routeParams.empId) {
$scope.Id = $routeParams.empId;
$http.get('api/employee/GetEmployee/' + $scope.Id).success(function (data) {
$scope.FirstName = data.FirstName;
$scope.LastName = data.LastName;
$scope.Address = data.Address;
$scope.Salary = data.Salary;
$scope.DOB = data.DOB
//$scope.DepartmentID = data.DepartmentID
});
}
//Update the employee records
$scope.Update = function () {
debugger;
var employeeData = {
EmployeeID: $scope.Id,
FirstName: $scope.FirstName,
LastName: $scope.LastName,
Address: $scope.Address,
Salary: $scope.Salary,
DOB: $scope.DOB
//DepartmentID: $scope.DepartmentID
};
if ($scope.Id > 0) {
$http.put("api/employee/UpdateEmployee", employeeData).success(function (data) {
$location.path('/EmployeeList');
}).error(function (data) {
console.log(data);
$scope.error = "Something wrong when adding updating employee " + data.ExceptionMessage;
});
}
}
//Delete the selected employee from the list
$scope.Delete = function () {
if ($scope.Id > 0) {
$http.delete("api/employee/DeleteEmployee/" + $scope.Id).success(function (data) {
$location.path('/EmployeeList');
}).error(function (data) {
console.log(data);
$scope.error = "Something wrong when adding Deleting employee " + data.ExceptionMessage;
});
}
}
}]);
So, we have done mostly everything besides creating template file. So, first I will create the template file for showing the list of employee.
Employee List
To see the employee list, need to create EmployeeList.html page.
<a href="#/AddEmployee" class="btn btn-primary btn-xs" >Add New Employee</a>
<br /><br />
<div class="table-responsive">
<table id="mytable" class="table table-bordred table-striped">
<thead>
<th>ID</th>
<th>First Name</th>
<th>Last Name</th>
<th>Address</th>
<th>Salary</th>
<th>DOB</th>
<!--<th>Department</th>-->
<th>Edit</th>
<th>Delete</th>
</thead>
<tbody>
<tr ng-repeat="item in ListOfEmployee">
<td>{{item.EmployeeID}}</td>
<td>{{item.FirstName}}</td>
<td>{{item.LastName}}</td>
<td>{{item.Address}}</td>
<td>{{item.Salary}}</td>
<td>{{item.DOB}}</td>
<!--<td>{{item.DepartmentName}}</td>-->
<td><a class="btn btn-primary btn-xs" href="#/EditEmployee/{{item.EmployeeID}}">
<span class="glyphicon glyphicon-pencil"></span></a></td>
<td><a class="btn btn-danger btn-xs" href="#/DeleteEmployee/{{item.EmployeeID}}">
<span class="glyphicon glyphicon-trash"></span></a></td>
</tr>
</tbody>
</table>
</div>
Now it is time to run the project and see how data will be displayed with angular js app. To run the application, just press F5 and change the url as "http://localhost:56983/#/EmployeeList". When application will run, output will be like as below image.
Add Employee
To add new employe with Angular JS application, just need to create one more template page with name "AddEmployee.html" as following.
<div id="add" tabindex="-1" role="dialog" aria-labelledby="add-modal-label">
<div class="modal-dialog" role="document">
<div class="modal-content">
<form class="form-horizontal" id="add-form">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-label="Close"><span aria-hidden="true">×</span></button>
<h4 class="modal-title" id="add-modal-label">Add new Employee</h4>
</div>
<div class="modal-body">
<div class="form-group">
<label for="add-firstname" class="col-sm-2 control-label">Firstname</label>
<div class="col-sm-10">
<input type="text" class="form-control" id="add-firstname" name="firstname" placeholder="Firstname" ng-model="FirstName" required>
</div>
</div>
<div class="form-group">
<label for="add-lastname" class="col-sm-2 control-label">LastName</label>
<div class="col-sm-10">
<input type="text" class="form-control" id="add-lastname" name="lastName" placeholder="LastName" ng-model="LastName" required>
</div>
</div>
<div class="form-group">
<label for="add-address" class="col-sm-2 control-label">Address</label>
<div class="col-sm-10">
<input type="text" class="form-control" id="add-address" name="address" placeholder="Address" ng-model="Address">
</div>
</div>
<div class="form-group">
<label for="add-salary" class="col-sm-2 control-label">Salary</label>
<div class="col-sm-10">
<input type="text" class="form-control" id="add-salary" name="mobile" placeholder="Salary" ng-model="Salary" required>
</div>
</div>
<div class="form-group">
<label for="add-dob" class="col-sm-2 control-label">DOB</label>
<div class="col-sm-10">
<input type="text" class="form-control" id="add-dob" name="mobile" placeholder="DOB" ng-model="DOB" required> [ex: 1990-01-21]
</div>
</div>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-default" data-dismiss="modal" ng-click="Close()">Close</button>
<button type="submit" class="btn btn-primary" ng-click="Add()">Save changes</button>
</div>
</form>
</div>
</div>
</div>
Now just click on the "Add New Employee" button or change the url as like "http://localhost:56983/#/AddEmployee". It will show the page with some control where you can enter the required details for employee. When you click on Save changes then it will add the new employee.
Update Employee
From the employee list page, there is button to edit/update the employee list. To click on any, you can update the respected details for that employee. Create the EditEmployee.html page as following.
<div id="edit" aria-labelledby="edit" aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<form class="form-horizontal" id="edit-form">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-label="Close"><span aria-hidden="true">×</span></button>
<h4 class="modal-title" id="edit-modal-label">Update Employee</h4>
</div>
<div class="modal-body">
<div class="form-group">
<label for="edit-firstname" class="col-sm-2 control-label">Firstname</label>
<div class="col-sm-10">
<input type="text" class="form-control" id="edit-firstname" name="firstname" placeholder="Firstname" ng-model="FirstName" required>
</div>
</div>
<div class="form-group">
<label for="edit-lastname" class="col-sm-2 control-label">LastName</label>
<div class="col-sm-10">
<input type="text" class="form-control" id="edit-lastname" name="lastName" placeholder="LastName" ng-model="LastName">
</div>
</div>
<div class="form-group">
<label for="edit-address" class="col-sm-2 control-label">Address</label>
<div class="col-sm-10">
<input type="text" class="form-control" id="edit-address" name="address" placeholder="Address" ng-model="Address">
</div>
</div>
<div class="form-group">
<label for="edit-salary" class="col-sm-2 control-label">Salary</label>
<div class="col-sm-10">
<input type="text" class="form-control" id="edit-salary" name="mobile" placeholder="Salary" ng-model="Salary">
</div>
</div>
<div class="form-group">
<label for="edit-dob" class="col-sm-2 control-label">DOB</label>
<div class="col-sm-10">
<input type="text" class="form-control" id="edit-dob" name="mobile" placeholder="DOB" ng-model="DOB">
</div>
</div>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-default" ng-click="Close()">Close</button>
<button type="submit" class="btn btn-primary" ng-click="Update()">Update</button>
</div>
</form>
</div>
<!-- /.modal-content -->
</div>
<!-- /.modal-dialog -->
</div>
and result will be displayed as like following
Delete Employee
To delete the employee, just click on delete button to delete respective employee. I have created DeleteEmployee.html page.
<div id="delete" ng-controller="EmployeeController">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-hidden="true"><span class="glyphicon glyphicon-remove" aria-hidden="true"></span></button>
<h4 class="modal-title custom_align" id="Heading">Delete this entry</h4>
</div>
<div class="modal-body">
<div class="alert alert-danger"><span class="glyphicon glyphicon-warning-sign"></span> Are you sure you want to delete Record for <b>{{FirstName + " "+LastName}}</b>?</div>
</div>
<div class="modal-footer ">
<button type="submit" class="btn btn-success" ng-click="Delete()"><span class="glyphicon glyphicon-ok-sign"></span> Yes</button>
<button type="button" class="btn btn-default" ng-click="Close()"><span class="glyphicon glyphicon-remove"></span> No</button>
</div>
</div>
<!-- /.modal-content -->
</div>
<!-- /.modal-dialog -->
</div>
When you will click on delete button, it will open a page where it will ask to confirmation for delete.
Conclusion:
So, today we have learned how to create a Web API and how to create an Angular JS and perform CRUD operations.
I hope this post will help you. Please put your feedback using comment which helps me to improve myself for next post. If you have any doubts please ask your doubts or query in the comment section and If you like this post, please share it with your friends. Thanks
Posted Comments :

Mukesh Kumar Author Posted : 8 Years Ago
Hi, Thanks for your valuable comment, I want to confirm here that AngularJs is a client side javascript framework which is based on MVC framework to create Single Page Application [SPA]. It is open source and created by Google. It is fully structured framework. There is no need to know the scripting language like Javascript if you are going to work with AngularJS. Basically it is used to create Client Side Single Page Application. As some developers say that it is a library but it’s framework for creating the dynamic web pages.
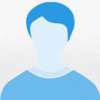
apurv1993 Posted : 8 Years Ago
using this code insert and display work properly,but when i click on delete icon i get particular id in url but can't get the delete confirmation modal.
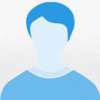
devarajan Posted : 8 Years Ago
Hi Mukesh, Thanks for your posting. My doubt is want to connect sql server?
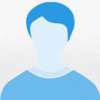
Devarajan Posted : 8 Years Ago
Hi Mukesh, Great job keep rock, I got Output.
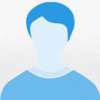
kumar Posted : 6 Years Ago
hello sir, nice one how to implemented login and registration page same respective like same angularjs and webapi using login and registration page sir can you share with me
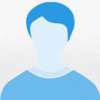
LKSharma Posted : 6 Years Ago
Why you removed that department module? can you pls send me the code with department in seprate table.
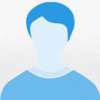
Gautam Sharma Posted : 6 Years Ago
Hi Mukesh Sir nice post I read the whole post but the only problem I am facing is that my sql server is not responding and I am unable to connect to it please guide me how to do it
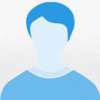
Atul Posted : 6 Years Ago
Thanks and great tutorial. However when i run the code for datatable having relational ( one to one / One to Many) to other datatable than its not showing any data in the table. Please help
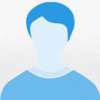
Yasmean Posted : 5 Years Ago
I am not able to download the code from this page. It navigates to this page "https://docs.microsoft.com/en-us/samples/browse/?redirectedfrom=MSDN-samples" Could you share me the link to download this code?
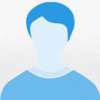
Khushboo Posted : 5 Years Ago
Nice tutorial but I can't download this project nd I have a question about database. When I'm adding the data it is not show the EmployeeList. What should I do?
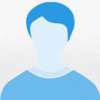
chandu Posted : 3 Years Ago
hi sir u have been good expalian.my req can u please make a video in youtube
@MM Posted : 8 Years Ago
Sir, i am new to .Net i know basics of Javascript. Can i learn Angular Js without going further into Javascript??