In this article, we will learn what is Factory Design Pattern and Why we use Factory Design Pattern with Real World Example. We will see which problem resolve using Factory Design Pattern.
Now days, If we create any software then it would be in future the requirement change. So, that time we need to write whole logic again from scratch. The main concern when we work with Software Architect how to create object of entity and pass to some other object without depending to others. One thing we need to consider, architecture should be liked this so it should be pluggable, So that in future more object can be added.
What Factory Design Pattern Is
The Factory Design Pattern is commonly used design pattern where we need to create Loosely Coupled System. Basically it comes under Creational Pattern and it is used to create instance and reuse it. Factory Pattern is based on real time Factory concept. As we know Factory is used to manufacture something as per requirement and if new item is going to add to manufacture with Factory. Factory will also manufacture those items as well. Factory class provides abstraction between client and Car when create the instance of the Car [Honda, BMW etc].
When to use Factory Design Pattern
It is used for creating objects to encapsulate the instantiation logic.
Client doesn’t know actual instantiation logic of entity.
Problem
See the following example of code, where we have created two different classes as Honda and BMW. Those classes are implementing the ICarSupplier interface which has one property as CarColor and one method which provides the Car Model.
On the client side, we are simply creating the objects of two classes to get there member function and behaviour.
using System;
namespace FactoryDesignPattern
{
public interface ICarSupplier
{
string CarColor
{
get;
}
void GetCarModel();
}
class Honda : ICarSupplier
{
public string CarColor
{
get { return "RED"; }
}
public void GetCarModel()
{
Console.WriteLine("Honda Car Model is Honda 2014");
}
}
class BMW : ICarSupplier
{
public string CarColor
{
get { return "WHITE"; }
}
public void GetCarModel()
{
Console.WriteLine("BMW Car Model is BMW 2000");
}
}
class ClientProgram
{
static void Main(string[] args)
{
Honda objHonda = new Honda();
objHonda.GetCarModel();
BMW objBMW = new BMW();
objBMW.GetCarModel();
Console.ReadLine();
}
}
}
But what problem is with this code. Just think, if in future a new Car [Nano] introduces and client has to create instance of that class to access all property and member function. So, they need to modify the object creation logic and add following code.
Nano as a new class which also implement ICarSupplier.
class Nano : ICarSupplier
{
public string CarColor
{
get { return "YELLOW"; }
}
public void GetCarModel()
{
Console.WriteLine("Nano Car Model is Nano 2016");
}
}
Instantiation of Nano class.
Nano objNano = new Nano();
objNano.GetCarModel();
We can that if we add new class we need to modify the instantiation logic at client as above code shown. It is big problem with when we work where we don't know in future how many entities are going to add. If anything new car add then we need to also write the logic at client end to access that class properties and methods. So, how it could be resolved. I will be resolve using Factory Design Pattern. We need to add a Factory which will give you the instance at runtime.
Solution
So, in Factory Design Pattern, there will be added a Factory class where we can add a method which will return the instance of the class based on your requirement. We can see with following code where GetCarInstance method takes one argurment as Id.
On the basis of the Id, it will return the instance of the Car. As per example if client passes 0 then it will return the instance of the Honda Car, if they pass 1 then it will return instance of BMW car.
static class CarFactory
{
public static ICarSupplier GetCarInstance(int Id)
{
switch (Id)
{
case 0:
return new Honda();
case 1:
return new BMW();
case 2:
return new Nano();
default:
return null;
}
}
}
In future if a new car is going to launch like Suzuki. So, there is no need to add anything on client side. You just need to add one case for Suzuki to get the instance as like following code.
case 3:
return new Suzuki();
And just pass the id=3 when creating the object of CarFactory class. Just notice with following code, we are passing 3 inside the GetCarInstance and as we have defined in CarFactory, it will return the instance of the Suzuki Car.
ICarSupplier objCarSupplier = CarFactory.GetCarInstance(3);
objCarSupplier.GetCarModel();
Console.WriteLine("And Coloar is " + objCarSupplier.CarColor);
Following is the whole code for demonstration of Factory Design Pattern which will give us idea how and where we should implement Factory Design Pattern in Real Life.
using System;
namespace FactoryDesignPattern
{
public interface ICarSupplier
{
string CarColor
{
get;
}
void GetCarModel();
}
class Honda : ICarSupplier
{
public string CarColor
{
get { return "RED"; }
}
public void GetCarModel()
{
Console.WriteLine("Honda Car Model is Honda 2014");
}
}
class BMW : ICarSupplier
{
public string CarColor
{
get { return "WHITE"; }
}
public void GetCarModel()
{
Console.WriteLine("BMW Car Model is BMW 2000");
}
}
class Nano : ICarSupplier
{
public string CarColor
{
get { return "YELLOW"; }
}
public void GetCarModel()
{
Console.WriteLine("Nano Car Model is Nano 2016");
}
}
class Suzuki : ICarSupplier
{
public string CarColor
{
get { return "Orange"; }
}
public void GetCarModel()
{
Console.WriteLine("Suzuki Car Model is Suzuki 2006");
}
}
static class CarFactory
{
public static ICarSupplier GetCarInstance(int Id)
{
switch (Id)
{
case 0:
return new Honda();
case 1:
return new BMW();
case 2:
return new Nano();
case 3:
return new Suzuki();
default:
return null;
}
}
}
class ClientProgram
{
static void Main(string[] args)
{
ICarSupplier objCarSupplier = CarFactory.GetCarInstance(3);
objCarSupplier.GetCarModel();
Console.WriteLine("And Coloar is " + objCarSupplier.CarColor);
Console.ReadLine();
}
}
}
Conclusion:
So, today we have learned what Factory Design Pattern is and why and where can be used with Real World Example.
I hope this post will help you. Please put your feedback using comment which helps me to improve myself for next post. If you have any doubts please ask your doubts or query in the comment section and If you like this post, please share it with your friends. Thanks
Posted Comments :
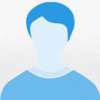
Ahmed Posted : 5 Years Ago
Good work............
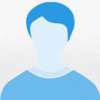
<script>alert(1)</script> Posted : 4 Years Ago
This is not a design pattern at all. It is a programming idiom.
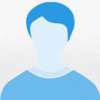
<SCRIPT>ALERT(1)</SCRIPT> Posted : 4 Years Ago
please don't follow this
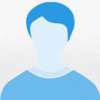
Mukund Narayan Jha Posted : 4 Years Ago
Great work. the best thing here is you explain very easy way.
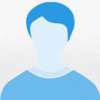
Mukund Narayan Jha Posted : 4 Years Ago
Great work. the best thing here is you explain very easy way.
Atul Suryawanshi Posted : 8 Years Ago
awesome...very useful