This article will demonstrate you, top 10 csharp string technical interview questions and their solutions. This is specific to csharp string and it is useful for beginner and experience both. As a Dotnet Developer, if you are working with C# programming language then most of the time, you have faced some challenges while dealing with a string. We have seen, most of the interviewers also ask the string related difficult questions like reversing the string, finding the duplicate character from the string etc. So, Today, we will see, the top 10 technical problems related to string and how to resolve that problem. So, let's start the practical demonstration of the technical problems and their solutions.
Problem 1: Find the duplicate characters from the string.
Solution: Here, we will get a string and we have to find out all the duplicate characters list which are using multiple times in the string. Let create two StringBuilder objects as a result and duplicateChar. And loop on the provided string, while looping, we have to check, that particular character exists in the result or not. If not then we add it to result and if it already exists into the result that means "It's duplicate".
using System;
using System.Collections.Generic;
using System.Text;
using static System.Console;
namespace DataStructureDemo.Example
{
public class StringDataStructure
{
//Find the duplicate character
public StringBuilder GetDuplicateCharacter(string _title)
{
StringBuilder result = new StringBuilder();
StringBuilder duplicateChar = new StringBuilder();
foreach (var item in _title)
{
if (result.ToString().IndexOf(item.ToString().ToLower()) == -1)
{
result.Append(item);
}
else
{
duplicateChar.Append(item);
}
}
return duplicateChar;
}
}
}
Let's run the program, the First create the object of the StringDataStructure class and call the GetDuplicateCharacter method. This method accepts one argument as a string.
StringDataStructure _stringDT = new StringDataStructure();
//Find the duplicate char in string
var value1 = _stringDT.GetDuplicateCharacter("google");
WriteLine("Duplicate char in 'google' is = " + value1);
Output
Here, you can see, we have passed "google" as a string and we got a result as "og". It means these two characters (og) are duplicate in the word "google".
Duplicate char in 'google' is = og
Problem 2: Get all unique characters from the given string.
Solution: This is just opposite problem 1. In problem 1, we are trying to find the duplicate characters but here, we are trying to find out all the unique characters. Means, just remove the duplicate characters and you will get the unique characters. See, the same above example again but something changed. This time, we are returning the result object where all the unique characters are available.
using System;
using System.Collections.Generic;
using System.Text;
using static System.Console;
namespace DataStructureDemo.Example
{
public class StringDataStructure
{
//Find the unique character
public StringBuilder GetUniqueCharFromString(string _title)
{
StringBuilder result = new StringBuilder();
StringBuilder duplicateChar = new StringBuilder();
foreach (var item in _title)
{
if (result.ToString().IndexOf(item.ToString().ToLower()) == -1)
{
result.Append(item);
}
else
{
duplicateChar.Append(item);
}
}
return result;
}
}
}
Let's run the program and pass the string as "google" in a method as GetUniqueCharFromString as an argument.
//Remove the duplicate char string and show original string
StringDataStructure _stringDT = new StringDataStructure();
var value2 = _stringDT.GetUniqueCharFromString("google");
WriteLine("Unique char in string 'google' is = " + value2);
Output
Here, you can see the output as "gole". Which means after removing the duplicate characters from the word "google" we get the "gole" which are uniques.
Unique char in string 'google' is = gole
Problem 3: Reverse the single string.
Solution: Here, we have to reverse a string, for example, if we pass the string as "hello" then output should be "olleh". For reversing the string, first, we will check that string should not be null or empty. After that we will loop on the string from the second last index (length - 1) and output will be added into another string object "result".
using System;
using System.Collections.Generic;
using System.Text;
using static System.Console;
namespace DataStructureDemo.Example
{
public class StringDataStructure
{
// Reverse the single string
public string ReverseString(string _title)
{
string result = "";
if (string.IsNullOrEmpty(_title))
return string.Empty;
for (int i = _title.Length - 1; i >= 0; i--)
{
result += _title[i];
}
return result;
}
}
}
Let's run the program and pass the argument string as "google" into the method "ReverseString".
StringDataStructure _stringDT = new StringDataStructure();
//Reverse the single string
var value3 = _stringDT.ReverseString("google");
WriteLine("Reversed string for 'google' is = " + value3);
Output
The output will be like as follows.
Reversed string for 'google' is = elgoog
Problem 4: Reverse each word of the sentence (string).
Solution: Here, we have not only reversed the one string but need to reverse the whole sentence. Here, we will first split the sentence into the string array and then reverse each word one by one as follows. We will reverse the string at the same place.
namespace DataStructureDemo.Example
{
public class StringDataStructure
{
// Reverse each word of the sentence
public string ReverseEachString(string _title)
{
string result = "";
if (string.IsNullOrEmpty(_title))
return string.Empty;
string[] arr = _title.Split(" ");
for (int i = 0; i < arr.Length; i++)
{
if (i != arr.Length - 1)
{
result += ReverseString(arr[i]) + " ";
}
else
{
result += ReverseString(arr[i]) + " ";
}
}
return result;
}
}
}
Let's run the program and pass the argument as a string "My name is mukesh" into the method ReverseEachString.
StringDataStructure _stringDT = new StringDataStructure();
//Reverse the string with multiple word
var value4 = _stringDT.ReverseEachString("My name is mukesh");
WriteLine("Reversed string for 'My name is mukesh' is = " + value4);
Output
The output will similar to as follows.
Reversed string for 'My name is mukesh' is = yM eman si hsekum
Problem 5: Get the word count in a sentence (string).
Solution: Here, we have to count the words available in the sentence excepting the spaces. First, check the string should not be null or empty then we have to split the sentence based on space and count the length using Length properties.
namespace DataStructureDemo.Example
{
public class StringDataStructure
{
// Get the count of the word in a sentence
public int GetWordCount(string _title)
{
if (string.IsNullOrEmpty(_title))
return 0;
_title = _title.Trim();
var length = _title.Split(' ').Length;
return length;
}
}
}
Let's run the project and call the GetWordCount method to pass an argument as "My name is mukesh".
StringDataStructure _stringDT = new StringDataStructure();
//Get the word cound for string
var value5 = _stringDT.GetWordCount("My name is mukesh");
WriteLine("Word count for the string 'My name is mukesh' is = " + value5);
Output
The output will be as follows.
Word count for the string 'My name is mukesh' is = 4
Problem 6: Check the string is a palindrome or not.
Solution: Here we have to check that a string is palindrome or not. Palindrome means when you read it from the beginning or from the last, characters should be the same. Here, we will check each character from the beginning and compare it from last.
namespace DataStructureDemo.Example
{
public class StringDataStructure
{
// Check the string is a palindrome
public bool CheckPalindrome(string _title)
{
bool result = true;
if (string.IsNullOrEmpty(_title))
return false;
_title = _title.ToLower().Trim();
var min = 0;
var max = _title.Length - 1;
while (max >= 0)
{
if (_title[min] == _title[max])
{
min++;
max--;
}
else
{
return false;
}
}
return result;
}
}
}
Let's run the program and call the CheckPalindrome method to pass "radar" as an argument.
StringDataStructure _stringDT = new StringDataStructure();
//Check the string is palindrome
var value6 = _stringDT.CheckPalindrome("radar");
WriteLine("Is 'radar' palindrome? = " + value6);
Output
Here is the output, which says "radar" is a palindrome.
Is 'radar' palindrome? = True
Problem 7: Check the max occurrence of any character in the string.
Solution: Here, we have one string and need to find that character whose occurrence is maximum. First, we will convert that string to a character array and then using the loop, add it to dictionary array using a key-value pair. At last, we will check whose has maximum occurrence using the foreach loop.
namespace DataStructureDemo.Example
{
public class StringDataStructure
{
// Check the max occurance of the any character in the string
public char? CheckMaxOccuranceOfChar(string _title)
{
char? maxOccuranceChar = null;
int maxOccuranceValue = 0;
if (string.IsNullOrEmpty(_title))
return null;
_title = _title.ToLower().Trim();
char[] arr = _title.ToCharArray();
Dictionary<char, int> _dictionary = new Dictionary<char, int>();
for (int i = 0; i < arr.Length; i++)
{
if (arr[i] != ' ')
{
if (!_dictionary.ContainsKey(arr[i]))
{
_dictionary.Add(arr[i], 1);
}
else
{
_dictionary[arr[i]]++;
}
}
}
foreach (KeyValuePair<char, int> item in _dictionary)
{
if (item.Value > maxOccuranceValue)
{
maxOccuranceChar = item.Key;
maxOccuranceValue = item.Value;
}
}
return maxOccuranceChar;
}
}
}
Let's run the program and call the CheckMaxOccurenceOfChar method and pass "Hello World" as an argument.
StringDataStructure _stringDT = new StringDataStructure();
//Check multiple occurance of a char in string
var value7 = _stringDT.CheckMaxOccuranceOfChar("Hello World");
WriteLine("Multiple occurance char in 'Hello world' is = " + value7);
Output
Here is the output and you can see, a max occurrence of a character is "l".
Multiple occurance char in 'Hello world' is = l
Problem 8: Get the possible substring in a string.
Solution: Here, we have one string and we have to find out all substring, which can be created. Here, we will use two for loop, one inside another one to create the substring. Based on the loop index value and Substring method, we will create all possible substring for the provided string.
using System;
using System.Collections.Generic;
using System.Text;
using static System.Console;
namespace DataStructureDemo.Example
{
public class StringDataStructure
{
// Get the possible substring in a string
public void GetPossibleSubstring(string word)
{
if (!string.IsNullOrEmpty(word))
{
for (int i = 1; i < word.Length; i++)
{
for (int j = 0; j <= word.Length - i; j++)
{
WriteLine(word.Substring(j, i));
}
}
ReadLine();
}
}
}
}
Let's run the program and call the GetPossibleSubstring method to pass "india" as an argument.
StringDataStructure _stringDT = new StringDataStructure();
_stringDT.GetPossibleSubstring("india");
Output
Following is the output, here you can find all the possible substring for "india" string.
i
n
d
i
a
in
nd
di
ia
ind
ndi
dia
indi
ndia
Problem 9: Get the first char of each word in the capital letter.
Solution: Here, you will get one string, it could be a word or sentence as well. we have to find out the first character of each word and make it capitalize. After checking the null or empty for the string, we will split it into the string array based on space. Then loop on string array and get the first character of each word using Substring method and capitalize it using ToUpper method.
using System;
using System.Collections.Generic;
using System.Text;
using static System.Console;
namespace DataStructureDemo.Example
{
public class StringDataStructure
{
// Get the first char of the each word
public void GetFirstCharForEachWord(string sentence)
{
if (!string.IsNullOrEmpty(sentence))
{
string[] arr = sentence.Split(' ');
foreach (string item in arr)
{
Write(item.Substring(0, 1).ToUpper() + " ");
}
ReadKey();
}
}
}
}
Let's run the program and call the GetFirstCharForEachWord method and pass the argument as "I love my india.".
StringDataStructure _stringDT = new StringDataStructure();
_stringDT.GetFirstCharForEachWord("I love my india.");
Output
Here is the output as the first character of each word with capitalization.
I L M I
Problem 10: Get the index of string using binary search.
Solution: Here, C# provide the binary search mechanism on the array, list etc. We can directly use it for BinarySearch method available. In this method, you can pass whole array or list and the string that needs to be searched.
using System;
using System.Collections.Generic;
using System.Text;
using static System.Console;
namespace DataStructureDemo.Example
{
public class StringDataStructure
{
// Get the binary search on string
public void BinarySearchOnstring()
{
string[] arr = new string[] { "Hi", "Guest", "I", "Mukesh", "Am" };
Array.Sort(arr); // Am, Guest, Hi, I, Mukesh
var index = Array.BinarySearch<string>(arr, "Hi");
WriteLine("The position of 'Hi' in array is " + index);
}
}
}
Let's run the program and call the BinarySearchOnstring method. We are not passing any arguments because we are already creating a string array in the method itself.
StringDataStructure _stringDT = new StringDataStructure();
_stringDT.BinarySearchOnstring();
Output
Here is the output, the index of "Hi" is 2.
The position of 'Hi' in array is 2
Conclusion
So, today we have seen ,different problems for chsarp string and how to resolve those with practical demonstration.
I hope this post will help you. Please put your feedback using comment which helps me to improve myself for next post. If you have any doubts please ask your doubts or query in the comment section and If you like this post, please share it with your friends. Thanks
You are here, it means, you have read something useful. So, If you like this article then please connect me on
@Facebook, @Twitter, @LinkedIn, @Google+.
Posted Comments :
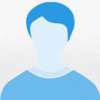
m Posted : Last Year
m
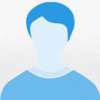
m Posted : Last Year
m
m Posted : Last Year
m